How to Improve SEO in Next.js: 8 Proven Best Practices 2025
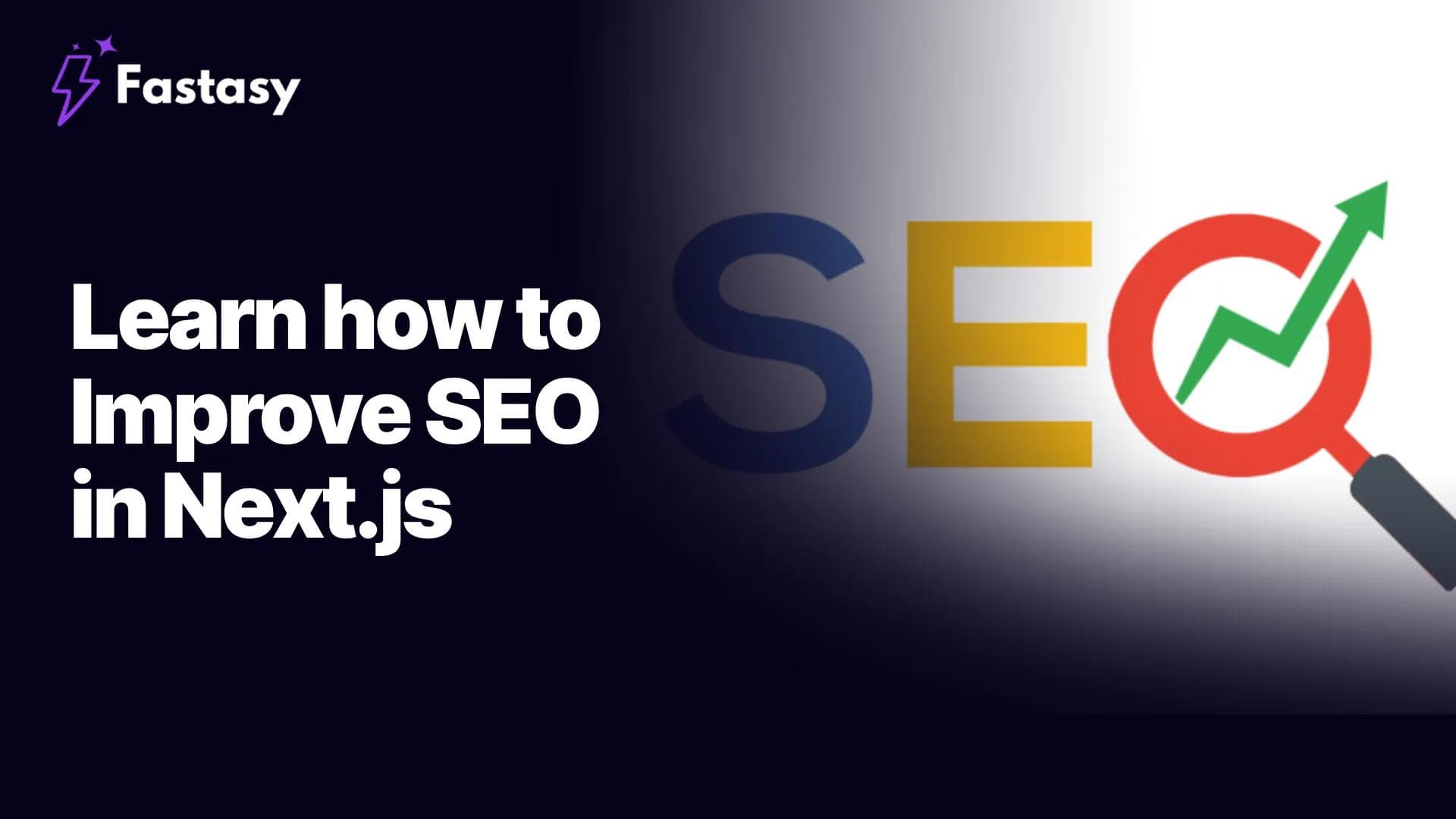
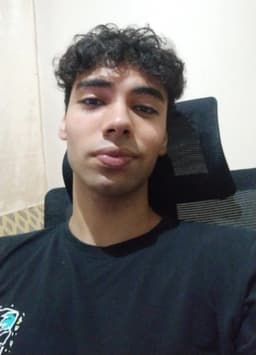
Look, if you're building websites with Next.js and you dont know how to improve SEO in Nextjs, No worries you're in the right place .
I'm Mostafa, and I've helped countless business websites improve their visibility in Google search results by implementing proper Next.js SEO strategies. Today, I'm sharing what's actually worked for me and my clients at Fastasy.
What is Next.js?
Next.js is a React framework that gives you the building blocks to create web applications. It handles the tooling and configuration needed for React, and provides additional structure, features, and optimizations.
But here's the thing most developers miss: Next.js isn't just about developer experience. It's actually built with SEO in mind from the ground up.
How Next.js Helps with SEO
Before we dive into specific tactics, you need to understand why Benefits of Next.js is a game-changer for SEO and why considered as one of the Best Front end Framework for Seo:
Server-side rendering (SSR) and static site generation (SSG) are built right in. This means search engines can crawl your content effortlessly, unlike traditional SPAs.
The latest Next.js 15 takes this even further with improved performance metrics that directly impact your search rankings.
Now let's get into what actually moves the needle.
Best Practices to Improve SEO in Next.js
1. Fonts Optimization
Web fonts can absolutely kill your Core Web Vitals if not handled properly. And Google is watching those metrics like a hawk.
Why it matters for SEO: Font loading can significantly impact Largest Contentful Paint (LCP) and Cumulative Layout Shift (CLS) metrics, which are direct ranking factors.
Here's how to optimize fonts in Next.js 15 with app router:
// app/layout.tsx
import { Inter } from 'next/font/google'
// Optimize fonts by using the built-in font loader
const inter = Inter({
subsets: ['latin'],
display: 'swap',
})
export default function RootLayout({
children,
}: {
children: React.ReactNode
}) {
return (
<html lang="en" className={inter.className}>
<body>{children}</body>
</html>
)
}
This code leverages Next.js 15's built-in font optimization system. It preloads font files, eliminates layout shifts, and implements font-display: swap for improved perceived performance.
2. Images Optimization
Images are often the heaviest elements on a webpage. Unoptimized images will tank your performance and push visitors away.
Why it matters for SEO: Large, unoptimized images hurt your Core Web Vitals and increase bounce rates, both of which damage your rankings.
You can simply optimize your images just by using next/image
component to all your images instead of normal html <image/>
tag
Here's how to properly optimize images in Next.js 15:
import Image from 'next/image'
export default function OptimizedImage() {
return (
<div>
<Image
src="/product-image.jpg"
alt="Detailed product description for SEO"
width={800}
height={600}
priority={true} // do it just for LCP images
/>
</div>
)
}
This code uses Next.js's Image component which automatically:
- Converts images to modern formats (WebP/AVIF)
- Lazy-loads images (unless priority is set)
- Prevents layout shifts with proper dimensions
- Serves responsive images based on device size
The priority
flag tells Next.js this image is above the fold and should be loaded immediately, which improves LCP for critical images.
3. Third-Party Scripts Optimization
Third-party scripts are often the silent killers of website performance. Analytics, ad trackers, chat widgets - they all add up.
Why it matters for SEO: Every script you add increases page load time and can block rendering, hurting your Core Web Vitals and search rankings.
Here's how to properly load scripts in Next.js 15:
import { GoogleAnalytics } from '@next/third-parties/google'
export default function RootLayout({
children,
}: {
children: React.ReactNode
}) {
return (
<html lang="en">
<body>{children}</body>
<GoogleAnalytics gaId="G-XYZ" />
</html>
)
}
@next/third-parties
is a library that provides a collection of components and utilities that improve the performance and developer experience of loading popular third-party libraries in your Next.js application
4. Meta Tags Optimization
Meta tags are one of the oldest SEO tactics, but they're still crucial. Next.js 15 makes implementing them incredibly simple.
Why it matters for SEO: Meta tags tell search engines what your page is about. Proper implementation improves click-through rates and helps search engines understand your content.
Here's how to implement meta tags in Next.js 15:
// app/page.tsx
import type { Metadata } from 'next'
export const metadata: Metadata = {
title: 'How to Improve SEO in Next.js | Fastasy',
description: 'Learn proven Next.js SEO best practices to boost your Google rankings. Optimize fonts, images, meta tags, and more for better visibility.',
keywords: 'Next.js SEO, improve SEO in Next.js, Next.js SEO best practices',
openGraph: {
title: 'How to Improve SEO in Next.js | Fastasy',
description: 'Learn proven Next.js SEO best practices to boost your Google rankings.',
url: 'https://www.fastasy.com/blog/how-to-improve-seo-in-nextjs',
siteName: 'Fastasy',
images: [
{
url: 'https://www.fastasy.com/images/nextjs-seo-guide.jpg',
width: 1200,
height: 630,
alt: 'Next.js SEO Guide',
},
],
locale: 'en_GB',
type: 'article',
},
twitter: {
card: 'summary_large_image',
title: 'How to Improve SEO in Next.js | Fastasy',
description: 'Learn proven Next.js SEO best practices to boost your Google rankings.',
images: ['https://www.fastasy.com/images/nextjs-seo-guide.jpg'],
},
}
export default function Page() {
return (
<div>
<h1>How to Improve SEO in Next.js</h1>
{/* Page content */}
</div>
)
}
This implementation uses Next.js 15's built-in metadata API, which automatically generates all necessary meta tags at build time, ensuring they're properly server-rendered.
5. Add Schema Markup
Schema markup is structured data that helps search engines understand your content better. It's essential for rich snippets and enhanced search results.
Why it matters for SEO: Schema markup can dramatically improve your SERP appearance, leading to higher click-through rates and better rankings.
Here's how to implement schema markup in Next.js 15:
// app/page.tsx
import { JsonLd } from 'react-schemaorg'
import Script from 'next/script'
export default function Page() {
return (
<div>
<h1>How to Improve SEO in Next.js</h1>
{/* Content */}
<Script
id="article-schema"
type="application/ld+json"
dangerouslySetInnerHTML={{
__html: JSON.stringify({
'@context': 'https://schema.org',
'@type': 'Article',
headline: 'How to Improve SEO in Next.js',
description: 'Learn proven Next.js SEO best practices to boost your Google rankings.',
image: 'https://www.fastasy.com/images/nextjs-seo-guide.jpg',
author: {
'@type': 'Person',
name: 'Mostafa',
},
publisher: {
'@type': 'Organization',
name: 'Fastasy',
logo: {
'@type': 'ImageObject',
url: 'https://www.fastasy.com/logo.png',
},
},
datePublished: '2025-03-18',
dateModified: '2025-03-18',
mainEntityOfPage: {
'@type': 'WebPage',
'@id': 'https://www.fastasy.com/blog/how-to-improve-seo-in-nextjs',
},
}),
}}
/>
)
}
This code adds Article schema to your page, which helps Google understand your content structure and potentially display rich snippets in search results.
6. Add a sitemap.xml File
A sitemap tells search engines which pages on your site should be crawled and indexed. It's essential for good SEO, especially for larger sites.
Why it matters for SEO: Sitemaps help search engines discover and index all your important pages, ensuring maximum visibility in search results.
Here's how to generate a sitemap in Next.js 15:
// app/sitemap.ts
import { MetadataRoute } from 'next'
export default function sitemap(): MetadataRoute.Sitemap {
return [
{
url: 'https://www.fastasy.com',
lastModified: new Date(),
changeFrequency: 'weekly',
priority: 1,
},
{
url: 'https://www.fastasy.com/about',
lastModified: new Date(),
changeFrequency: 'monthly',
priority: 0.8,
},
{
url: 'https://www.fastasy.com/blog/how-to-improve-seo-in-nextjs',
lastModified: new Date(),
changeFrequency: 'weekly',
priority: 0.9,
},
// Add more pages as needed
]
}
Next.js 15 automatically generates a sitemap.xml file from this configuration, which is served at /sitemap.xml
. This ensures search engines can easily discover all your important pages.
7. Add a robots.txt File
A robots.txt file tells search engines which parts of your site they're allowed to crawl and index. It's a fundamental SEO element.
Why it matters for SEO: Proper robots.txt configuration ensures search engines focus on your important content and don't waste crawl budget on irrelevant pages.
Here's how to add a robots.txt file in Next.js 15:
// app/robots.ts
import { MetadataRoute } from 'next'
export default function robots(): MetadataRoute.Robots {
return {
rules: {
userAgent: '*',
allow: '/',
disallow: ['/private/', '/admin/'],
},
sitemap: 'https://www.fastasy.com/sitemap.xml',
}
}
This code automatically generates a robots.txt file that allows crawling of all public pages while blocking access to private areas. It also points search engines to your sitemap.
8. Implementing Internationalization (i18n)
If you're targeting multiple countries or languages, i18n is essential for SEO. Next.js 15 makes it straightforward to implement.
Why it matters for SEO: Proper internationalization ensures users in different regions see the right content, and search engines understand which language versions to show in results.
Personally I did use internationalization for a client that we translate his content 3 different languages French, English and Portuguese and we saw a huge difference in search traffic because the English Keywords in his niche was too competitive
Here's how to implement i18n in Next.js 15:
// middleware.ts
import { NextRequest, NextResponse } from 'next/server'
import { match } from '@formatjs/intl-localematcher'
import Negotiator from 'negotiator'
// List of supported locales
const locales = ['en', 'fr', 'de']
const defaultLocale = 'en'
// Get the preferred locale from the request
function getLocale(request: NextRequest) {
const headers = Object.fromEntries(request.headers)
const negotiator = new Negotiator({ headers })
const languages = negotiator.languages()
return match(languages, locales, defaultLocale)
}
export function middleware(request: NextRequest) {
// Get the pathname from the request
const pathname = request.nextUrl.pathname
// Check if the pathname is missing a locale
const pathnameHasLocale = locales.some(
locale => pathname.startsWith(`/${locale}/`) || pathname === `/${locale}`
)
if (pathnameHasLocale) return
// Redirect to the locale-specific path
const locale = getLocale(request)
const newUrl = new URL(`/${locale}${pathname}`, request.url)
return NextResponse.redirect(newUrl)
}
export const config = {
matcher: [
// Skip all internal paths
'/((?!api|_next/static|_next/image|favicon.ico).*)',
],
}
This middleware detects the user's preferred language and redirects them to the appropriate localized version of your site. It also ensures search engines understand the relationship between your different language versions.
Conclusion
Implementing these 8 Next.js SEO best practices will dramatically improve your visibility in search results. I've seen businesses go from page 10 to page 1 in Google by properly optimizing their Next.js sites.
At Fastasy, we've helped countless clients boost their traffic and conversions through these exact strategies. If you're looking to improve your SEO in Next.js, start with these practices and watch your rankings climb.
Remember, how to improve SEO in Next.js isn't just about technical implementations - it's about creating a seamless, fast user experience that both visitors and search engines will love.
Want to learn more about Next.js development? Check out our other guides on the benefits of Next.js and how Next.js compares to WordPress for SEO.
Frequently Asked Questions
Hi! 👋🏻 Need a website for your business?
- Slow Loading Speed
- Hard to Use on Phones
- Confusing Layout
- Messy Design
- Not Secure
- Doesn't show on Google
- Can't edit Website Content
- Quick to Load
- Mobile Friendly
- Easy Navigation
- Clean and Organized
- Secure and Safe
- Easy to Find on Google (SEO)
- Easy to edit Website Content
What are you waiting for?
Get a website that looks great and lets you update content easily no coding needed! Grow your business online with Fastasy
Get started