How CSS Works Behind the Scenes: Deep Dive into the Process
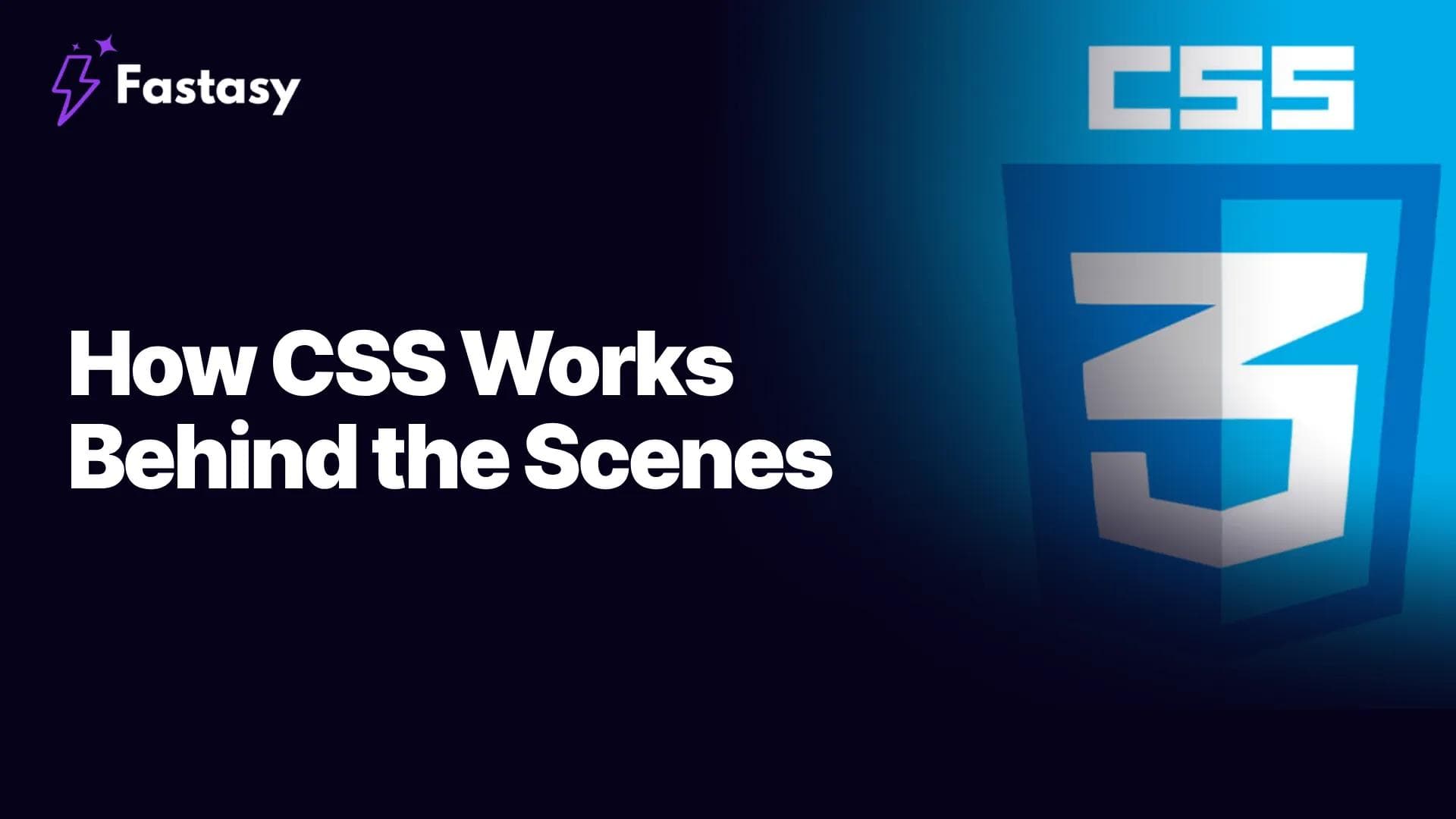
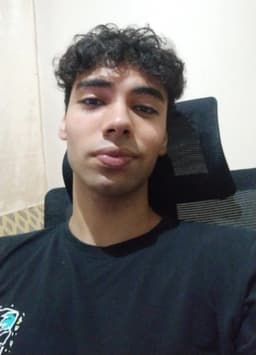
Ever wondered what actually happens when you link a CSS file to your HTML?
I spent years writing CSS without truly understanding what's happening under the hood.
Big mistake.
Once I understood how CSS works behind the scenes, my debugging skills shot through the roof, and my code got dramatically cleaner and faster.
Let me walk you through the entire process - no fluff, just practical insights on how CSS works behind the scenes that will make you a better developer.
Understanding How CSS Works Behind the Scenes: The Fundamentals
CSS might seem simple on the surface, but there's a complex machinery operating behind the scenes.
When you write a CSS rule, you're giving instructions to the browser's rendering engine.
But the browser doesn't just apply your styles immediately - it goes through a sophisticated process to interpret and apply those styles.
Understanding how CSS works behind the scenes gives you an edge when debugging style issues or optimizing performance.
You'll stop fighting the browser and start working with it.
Most performance issues I see beginners create come from not understanding how CSS works behind the scenes.
The Document Loading Journey
When a user visits your website, the browser first downloads your HTML document.
As the browser parses this HTML, it builds what's called the Document Object Model (DOM) - a tree-like representation of your page structure.
During this parsing process, when the browser encounters a link to a CSS file:
- It immediately requests that CSS file
- However, it doesn't wait for the CSS to download before continuing to parse the HTML
- This is why you'll sometimes see a flash of unstyled content (FOUC)
How CSS works behind the scenes during this initial loading phase directly impacts your page's perceived loading speed.
That's why critical CSS (loading only what's needed for above-the-fold content) has become such a popular optimization technique.
CSS Parsing Fundamentals
Once your CSS file is downloaded, the browser needs to make sense of it.
The parsing process breaks down your CSS into something the browser can work with:
- The browser tokenizes your CSS - breaking it into meaningful chunks
- It identifies selectors, properties, and values
- It validates the syntax (ignoring invalid rules)
- It normalizes values (converting colors to RGB, calculating relative units, etc.)
If you've ever wondered why a CSS rule isn't working, it could be getting ignored during this phase because of syntax errors.
Understanding how CSS works behind the scenes here helps you write more robust styles.
I've seen developers spend hours debugging CSS issues that stem from simple parsing problems.
The CSS Rule Processing
Each CSS rule has two main parts: a selector and one or more declarations.
The browser processes these very differently:
- Selectors determine which elements the rule applies to
- Declarations specify what styles should be applied
Behind the scenes, CSS processing is actually backwards from how we write it:
- The browser identifies all elements in the DOM
- For each element, it finds all selectors that match it
- Then it applies the relevant declarations
This is crucial to understand because it impacts performance - complex selectors cost more in processing time.
The more specific your selectors are, the more work the browser has to do behind the scenes.
The Cascade Mechanism Explained
The "C" in CSS stands for "Cascading" - and it's at the heart of how CSS works behind the scenes.
When multiple conflicting style rules target the same element, the browser needs to decide which one wins.
This cascade mechanism follows a strict hierarchy:
- Find all declarations that apply to the element
- Sort by origin and importance
- Sort by specificity
- Sort by order of appearance
Understanding this cascade is crucial for predictable styling.
I can't tell you how many times I've seen developers add unnecessary !important flags because they didn't understand how CSS works behind the scenes with the cascade.
Style Sources and Origin Priority
Your custom styles aren't the only ones the browser considers.
CSS comes from three main origins, and the browser prioritizes them in this order:
- User agent styles (the browser's default styles)
- User styles (custom settings applied by the user)
- Author styles (your CSS files)
Within each origin, inline styles take precedence over external stylesheets.
How CSS works behind the scenes with these different origins explains why sometimes you need to explicitly override certain styles.
This is why CSS resets became popular - they neutralize user agent styles to give you a clean slate.
The !important Flag and Importance Hierarchy
The !important flag changes everything about how CSS works behind the scenes.
When you add !important to a declaration, it jumps ahead in the cascade regardless of specificity or source order.
The actual processing order becomes:
- User agent normal declarations
- User normal declarations
- Author normal declarations
- Author !important declarations
- User !important declarations
- User agent !important declarations
Notice how user !important declarations outrank author !important declarations?
This ensures users with accessibility needs can override author styles.
Understanding how CSS works behind the scenes with !important helps you avoid overusing this powerful but potentially problematic feature.
Understanding Specificity Calculations
Specificity is a weight assigned to CSS selectors, and it's a fundamental part of how CSS works behind the scenes.
The browser calculates specificity as a four-part value (a,b,c,d):
- a: Inline styles (1 if present, 0 if not)
- b: Number of ID selectors
- c: Number of class selectors, attribute selectors, and pseudo-classes
- d: Number of element selectors and pseudo-elements
Higher values in earlier positions always win, regardless of values in later positions.
That's why a single ID selector (#header) will always override a selector with many classes (.navigation .list .item).
Understanding how CSS works behind the scenes with specificity calculations will save you countless debugging hours.
Source Order and Style Resolution
When all else is equal in terms of origin, importance, and specificity, the browser falls back to source order.
The last declaration encountered wins.
This is why:
p { color: red; }
p { color: blue; }
Results in blue text.
It's also why CSS frameworks place their styles before yours in the document - so your custom styles can easily override the framework defaults.
Understanding how CSS works behind the scenes with source order helps you structure your stylesheets more effectively.
Value Processing and Computation
Once the browser determines which declarations apply, it needs to calculate the final values.
This process is more complex than you might think:
- Specified values (what you wrote in your CSS)
- Computed values (after resolving relative units like em or %)
- Used values (after additional calculations based on layout)
- Actual values (after rounding to device pixels)
Understanding how CSS works behind the scenes during value computation helps explain why sometimes elements don't appear exactly as you specified.
For example, percentage-based margins and paddings are calculated based on the parent element's width, not its height.
Value Types and Property Inheritance
Not all CSS properties behave the same way behind the scenes.
Some properties naturally inherit from parent to child (like font-family or color).
Others don't inherit by default (like border or margin).
You can override this natural behavior with the inherit, initial, or unset keywords.
Understanding how CSS works behind the scenes with inheritance helps you write more concise CSS.
Instead of setting text color on every element, set it once on the body and let inheritance do its job.
Building the CSSOM
Just like HTML creates a DOM, CSS creates something called the CSS Object Model (CSSOM).
The CSSOM is a tree-like representation of all the styles that apply to your document.
Building the CSSOM is usually faster than building the DOM, but it's still a critical step in the rendering process.
How CSS works behind the scenes during CSSOM construction directly impacts your page's rendering performance.
Simpler stylesheets create simpler CSSOMs that browsers can process more quickly.
Combining DOM and CSSOM
Once both the DOM and CSSOM are ready, the browser combines them to create the render tree.
The render tree only includes nodes that will be visually displayed.
Elements with display: none don't appear in the render tree at all (unlike visibility: hidden, which still takes up space).
Understanding how CSS works behind the scenes during render tree construction explains why display: none completely removes elements from the layout flow.
This is powerful knowledge for creating toggle states without layout shifts.
The Visual Formatting Model
The visual formatting model is how CSS works behind the scenes to determine the exact layout of each element.
It considers:
- Box dimensions (from the box model)
- Box type (block, inline, inline-block)
- Positioning scheme (normal flow, float, absolute positioning)
- Relationships between elements
- External information (viewport size, images dimensions, etc.)
This is where your flexbox, grid, and positioning properties come into play.
Understanding the visual formatting model gives you precise control over your layouts.
Box Model Mechanics
The box model is fundamental to how CSS works behind the scenes.
Every element on your page is represented as a rectangular box with:
- Content area (where text and images appear)
- Padding (the space between content and border)
- Border (a line around the padding)
- Margin (the space between the border and other elements)
By default, width and height only control the content area.
This is why box-sizing: border-box became so popular - it makes width and height include padding and border, creating more intuitive sizing.
Understanding how CSS works behind the scenes with the box model prevents sizing surprises.
The Layout Calculation Process
Layout (sometimes called reflow) is one of the most intensive parts of how CSS works behind the scenes.
During this phase, the browser calculates the exact position and size of each element:
- It starts with the root element
- It works through the render tree, calculating positions and dimensions
- For elements with relative sizing, it needs to calculate their parent elements first
- It handles complex relationships like floats and absolutely positioned elements
Understanding how CSS works behind the scenes during layout helps you avoid performance pitfalls.
Properties that trigger layout (like width, height, or margin) are more expensive to change than properties that don't (like color or background-color).
The Paint and Compositing Phases
After layout comes painting - where the browser fills in pixels for each visible part of the elements.
The browser breaks the painting process into layers when possible:
- Individual layers are painted separately
- Then they're composited (combined) in the correct order
Some CSS properties (like box-shadow or border-radius) make the paint phase more complex.
Understanding how CSS works behind the scenes during painting explains why animations of certain properties perform better than others.
Properties that only affect compositing (like opacity or transform) are the cheapest to animate.
Performance Considerations
Now that you understand how CSS works behind the scenes, let's talk performance:
- Selector complexity affects CSSOM construction time
- Changes to layout properties force recalculation of element positions
- Paint properties can be expensive when they affect large areas
- Composite properties are cheapest to animate
When optimizing for performance:
- Minimize selector complexity
- Avoid unnecessary layout changes
- Use transform and opacity for animations when possible
- Split heavy animations onto their own layers with will-change
Understanding how CSS works behind the scenes makes you a performance-focused developer.
Conclusion
Understanding how CSS works behind the scenes isn't just academic knowledge - it's practical power.
It helps you:
- Debug styling issues more effectively
- Write more efficient CSS
- Create smoother animations and transitions
- Optimize your site's performance
The next time you're troubleshooting a CSS issue, think about which part of the behind-the-scenes process might be causing problems.
Are selectors conflicting? Is inheritance not working as expected? Are layout calculations causing performance issues?
Knowing how CSS works behind the scenes transforms you from someone who can write CSS into someone who truly understands it.
Frequently Asked Questions
Hi! 👋🏻 Need a website for your business?
- Slow Loading Speed
- Hard to Use on Phones
- Confusing Layout
- Messy Design
- Not Secure
- Doesn't show on Google
- Can't edit Website Content
- Quick to Load
- Mobile Friendly
- Easy Navigation
- Clean and Organized
- Secure and Safe
- Easy to Find on Google (SEO)
- Easy to edit Website Content
What are you waiting for?
Get a website that looks great and lets you update content easily no coding needed! Grow your business online with Fastasy
Get started