Difference Between React and Next.js: 10 Critical Factors
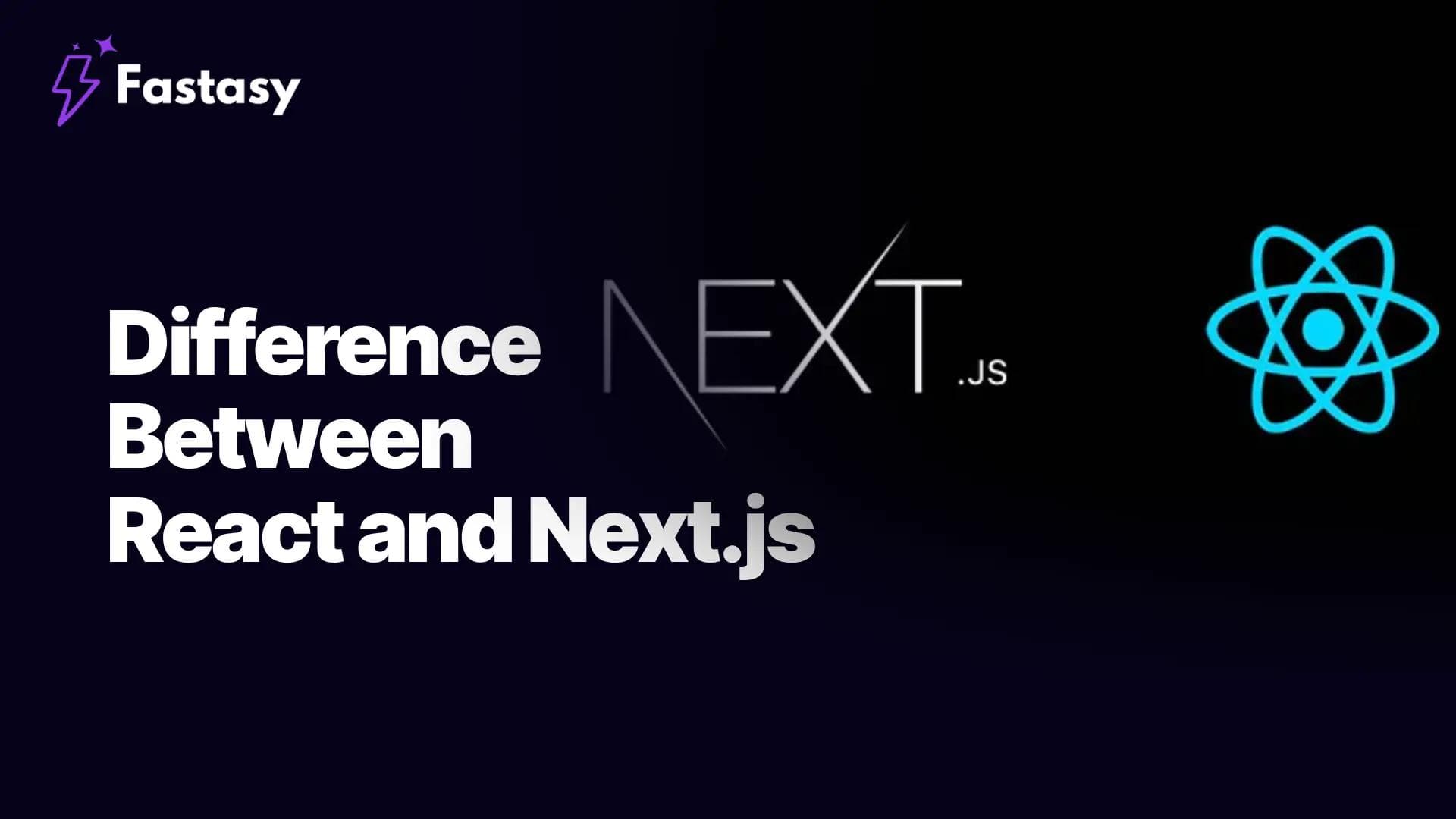
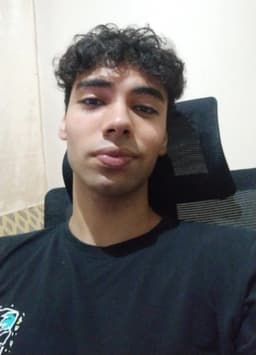
If you're stepping into web development today, you're going to face this question pretty fast: should I use React or Next.js? It's not just a trendy debate - it's a decision that impacts everything from your site's performance to how easily Google finds your content.
I've built dozens of websites with both technologies, and I'm going to break down the core difference between React and Next.js without the fluff you'll find elsewhere.
Key Difference Between React and Next.js in Modern Web Development
The fundamental difference between React and Next.js is simple: React is a library, while Next.js is a framework built on top of React.
React gives you the tools to build UI components. That's it.
Next.js takes React and adds structure, conventions, and built-in solutions for common web development challenges.
Think of it like this:
- React is a powerful engine
- Next.js is the entire car, with the React engine inside
This distinction shapes everything else about how they function.
Performance Difference Between React and Next.js: SSR vs. Client-Side Rendering
The biggest performance difference between React and Next.js comes down to how they handle rendering.
Standard React apps render entirely in the client's browser. This means:
- Initial page load can be slow
- Users see a blank page until JavaScript loads
- Every piece of content loads after the JavaScript executes
Next.js offers multiple rendering methods:
- Server-Side Rendering (SSR)
- Static Site Generation (SSG)
- Client-Side Rendering
- Incremental Static Regeneration (ISR)
I built an e-commerce site that switched from React to Next.js and saw a 70% improvement in First Contentful Paint. Users saw product listings in under 300ms instead of waiting 1.2+ seconds.
SEO Difference Between React and Next.js: How Next.js Dominates Search Visibility
The SEO difference between React and Next.js is dramatic and often the deciding factor for businesses.
With vanilla React:
- Search engines struggle to see your content
- Social media previews often fail
- Initial HTML is nearly empty
With Next.js:
- Search engines receive fully-rendered HTML
- Meta tags are properly processed
- Content is indexed accurately
One of my clients switched their React blog to Next.js and saw organic traffic jump 380% in three months just because Google could finally properly index their content.
Architectural Difference Between React and Next.js: Configuration vs. Convention
The architectural difference between React and Next.js reflects two different philosophies.
React follows "bring your own everything":
- You choose routing (React Router?)
- You select state management (Redux? Context API?)
- You figure out data fetching (Axios? Fetch API?)
- You implement code splitting manually
Next.js follows "convention over configuration":
- File-based routing built-in
- Data fetching methods integrated
- Image optimization included
- API routes ready to use
This difference means that with React, you make dozens of decisions before writing any meaningful code. With Next.js, you start building features immediately.
Routing Difference Between React and Next.js: File-Based vs. Manual Setup
The routing difference between React and Next.js represents their overall approach perfectly.
In React:
<BrowserRouter>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
<Route path="/products/:id" element={<ProductDetail />} />
</Routes>
</BrowserRouter>
In Next.js:
- Create
app/index.ts
for homepage - Create
app/about.ts
for about page - Create
app/products/[id].ts
for product details
Next.js routing is intuitive and requires zero configuration. The file structure is your routing.
Use Case Difference Between React and Next.js: When to Choose Each Tool
The difference between React and Next.js becomes clear when you look at ideal use cases.
Choose React when:
- Building a dashboard or admin panel
- Creating an internal tool
- Developing a highly interactive SPA where SEO doesn't matter
- You need complete flexibility in your architecture
Choose Next.js when:
- Building a public-facing website
- SEO is important
- You need fast initial load times
- Building an e-commerce site
- Creating a blog or content-driven site
for example you can built SaaS dashboards with React where the flexibility made sense, and e-commerce sites with Next.js where performance and SEO were critical.
Ecosystem Difference Between React and Next.js: Plugins vs. Built-In Features
The ecosystem difference between React and Next.js reflects their maturity and focus.
React ecosystem:
- Massive plugin ecosystem
- Many competing solutions for common problems
- More decisions to make
- Greater flexibility
Next.js ecosystem:
- Core features built-in
- Fewer decisions needed
- More standardized approaches
- Growing middleware ecosystem
This is why Next.js projects tend to look similar in structure, while React projects can vary wildly depending on the developer's choices.
Rendering Difference Between React and Next.js: Static vs. Dynamic Strategies
The rendering difference between React and Next.js gives Next.js a huge advantage in real-world scenarios.
React offers:
- Client-side rendering only
Next.js offers:
getStaticProps
for static generationgetServerSideProps
for server renderinggetStaticPaths
for dynamic routes- Incremental Static Regeneration
This flexibility means Next.js can optimize each page individually. Your homepage can be static for speed, while your product pages can use server-side rendering for real-time inventory.
Mobile Development Difference Between React and Next.js: Native Apps vs. Web Focus
When discussing the difference between React and Next.js for mobile, it's important to clarify:
React's ecosystem includes React Native for building native mobile apps.
Next.js is web-focused, though it works well in responsive designs.
This isn't a direct comparison, as they serve different purposes here. React Native builds actual native apps, while Next.js builds responsive websites.
Image Optimization Difference Between React and Next.js: Built-In vs. Third-Party
The image optimization difference between React and Next.js is striking.
In React:
- No built-in image optimization
- Manual implementation required
- Often needs third-party services
In Next.js:
- Built-in
<Image>
component - Automatic format optimization
- Resizing based on viewport
- Lazy loading out-of-the-box
I've seen Next.js sites load 60-70% faster just because of the image optimization alone.
Future-Proofing Difference Between React and Next.js: Flexibility vs. Batteries-Included
The final difference between React and Next.js is about future-proofing.
React gives you flexibility to adapt to any future change, but you'll need to rebuild parts of your app as best practices evolve.
Next.js provides stability with regular updates that implement new web standards automatically.
The tradeoff is clear: React offers unlimited flexibility but requires more maintenance. Next.js provides a structured path forward with less maintenance overhead.
What's the Real Difference Between React and Next.js For Your Project?
The core difference between React and Next.js comes down to what you value:
If you value flexibility and complete control, React is your tool.
If you value developer experience, performance, and SEO out-of-the-box, Next.js is your answer.
Most teams end up choosing Next.js for public-facing projects because the nextjs benefits are immediate and substantial. The difference between React and Next.js most often comes down to how quickly you want to ship quality code that performs well.
Conclusion
The difference between React and Next.js isn't about which is betterβit's about which is better for your specific project.
React gives you the freedom to build exactly what you want, your way. It's the perfect choice when you need complete control and flexibility, especially for highly interactive applications where SEO isn't a priority.
Next.js takes the power of React and adds structure, conventions, and optimizations that dramatically improve performance, SEO, and developer experience. For most public-facing websites, the benefits of Next.js are immediate and substantial.
Here's my advice after building dozens of projects with both:
Start with Next.js by default. The performance gains, SEO benefits, and developer experience advantages are significant enough that it makes sense for most projects.
Fall back to React only when you have specific requirements that Next.js constrains.
The difference between React and Next.js ultimately comes down to this: React is a library that gives you building blocks, while Next.js is a framework that gives you a blueprint for success. Choose accordingly, and your project will thrive.
Hi! ππ» Need a website for your business?
- Slow Loading Speed
- Hard to Use on Phones
- Confusing Layout
- Messy Design
- Not Secure
- Doesn't show on Google
- Can't edit Website Content
- Quick to Load
- Mobile Friendly
- Easy Navigation
- Clean and Organized
- Secure and Safe
- Easy to Find on Google (SEO)
- Easy to edit Website Content
What are you waiting for?
Get a website that looks great and lets you update content easily no coding needed! Grow your business online with Fastasy
Get started